Private and Different Layouts with React Router
I created a different template for React with React Router 4. The idea is:
- Code flexible able to manage many layouts.
- Possibility to choose a different layout for any component or a layout for a group of components.
- If a user is not logged in the URL’s that required a login, then show the public layout with a login form.
- If the user is logged and their wants see the public page (like about-us), the layout must be the public layout with the possibility to see the private web page if click in a private URL.
#
View Preview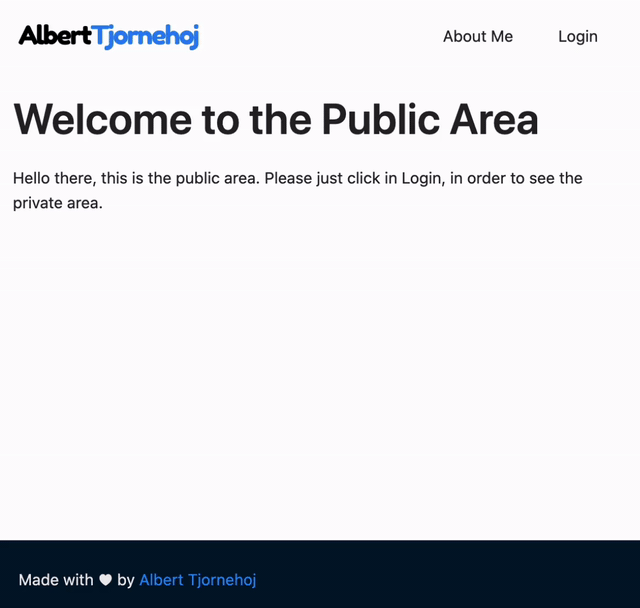
You can see this working live here
#
RoutesYou can create the routes in this way. You can create many files that you want:
#
Route TypesThere are three route types defined in the project.
private: private pages like profile, edit-profile, etc. If the user isn’t logged then must to show the login page.
public: public pages like about-us, contact, etc.
session: session pages like login and sign-up. If the user is logged then must to redirect to the private dashboard.
#
Routes TemplateIn this file you can define the routes, the template and the rights (public, private, session).
#
RouterIt define the route and call the Auth.
#
AuthVerify the rights and redirection.
You can download this code in my Github. I updated it, its working with the API code.