Basic example Laravel + GraphQL
Laravel GraphQL is a implementation of GraphQL with PHP for Laravel. Very easy to install and use. So the plan in this post is to create langs
query and see it in Graphiql.
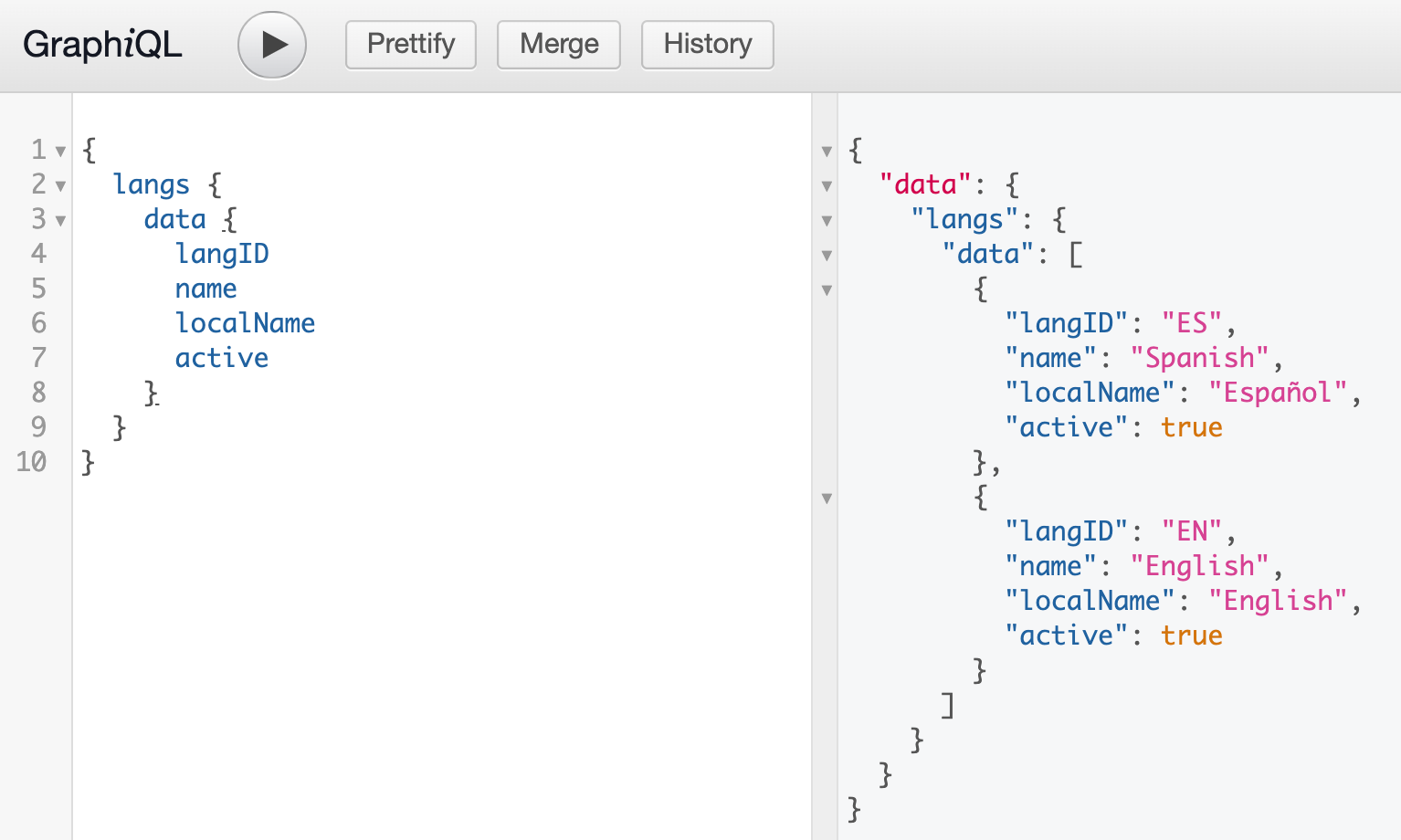
#
1. Install library:- Laravel GraphQL library.
#
2. Create the migration and the model:#
Create the MigrationI'm using PostgreSQL.
#
Create the ModelI created the class BaseModel to save the created_by
and updated_by
fields in the database.
#
3. Create the GraphQL Lang TypeThis file has the columns to return as the JSON variables. You can see whole file in github.
#
4. Create the queryThis is the basic example to return all the columns of the lang.lang
table.
#
5. Add the query and type to the config fileNow you can go to http://127.0.0.1:8000/graphiql/ and run the query and see the DB values in JSON format.
This is a very basic query. To make it better you have to work with the arguments and pagination. I already did it, so if you go to my laravel-graphql-api repository you can see it. If you would like to see it live please go here.