Private queries with Apollo Server and Express
I other post I was using a private and public schema to return 401 http status. But I found a better solution to change the response http status from the server.
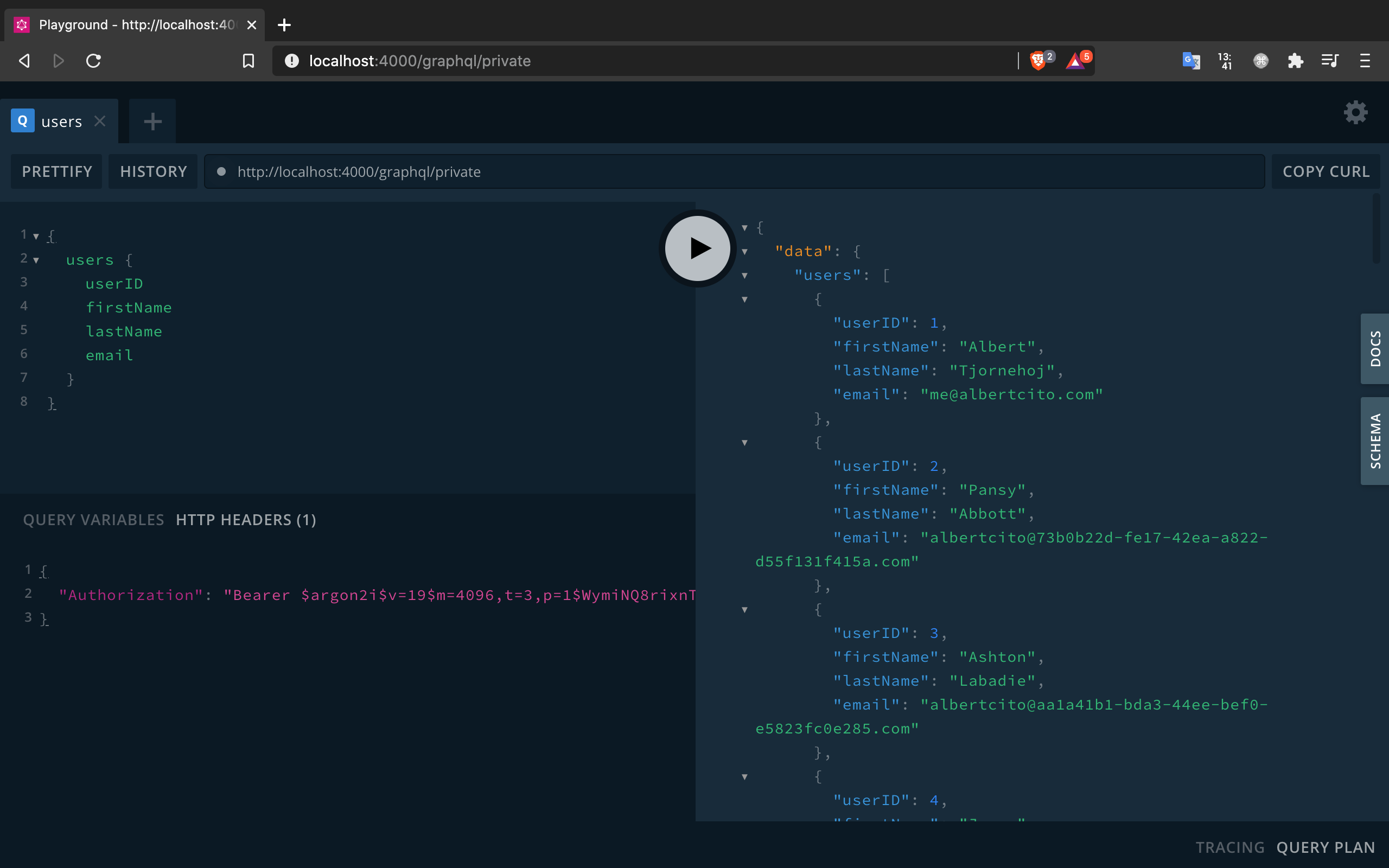
Apollo Server query with authenticate user
#
Create Exception#
Create Apollo Middleware#
Create query only access for authenticated users#
Intercept Error in global handle exceptionSo the ErrorHandlePlugin
will be the function to determine if the server response http status.
#
Add logic to Apollo ServerYou can see the code in my repo.