Authentication Laravel + GraphQL
In my previous post I published a basic example with Laravel + GraphQL. The current post is to create the login query.
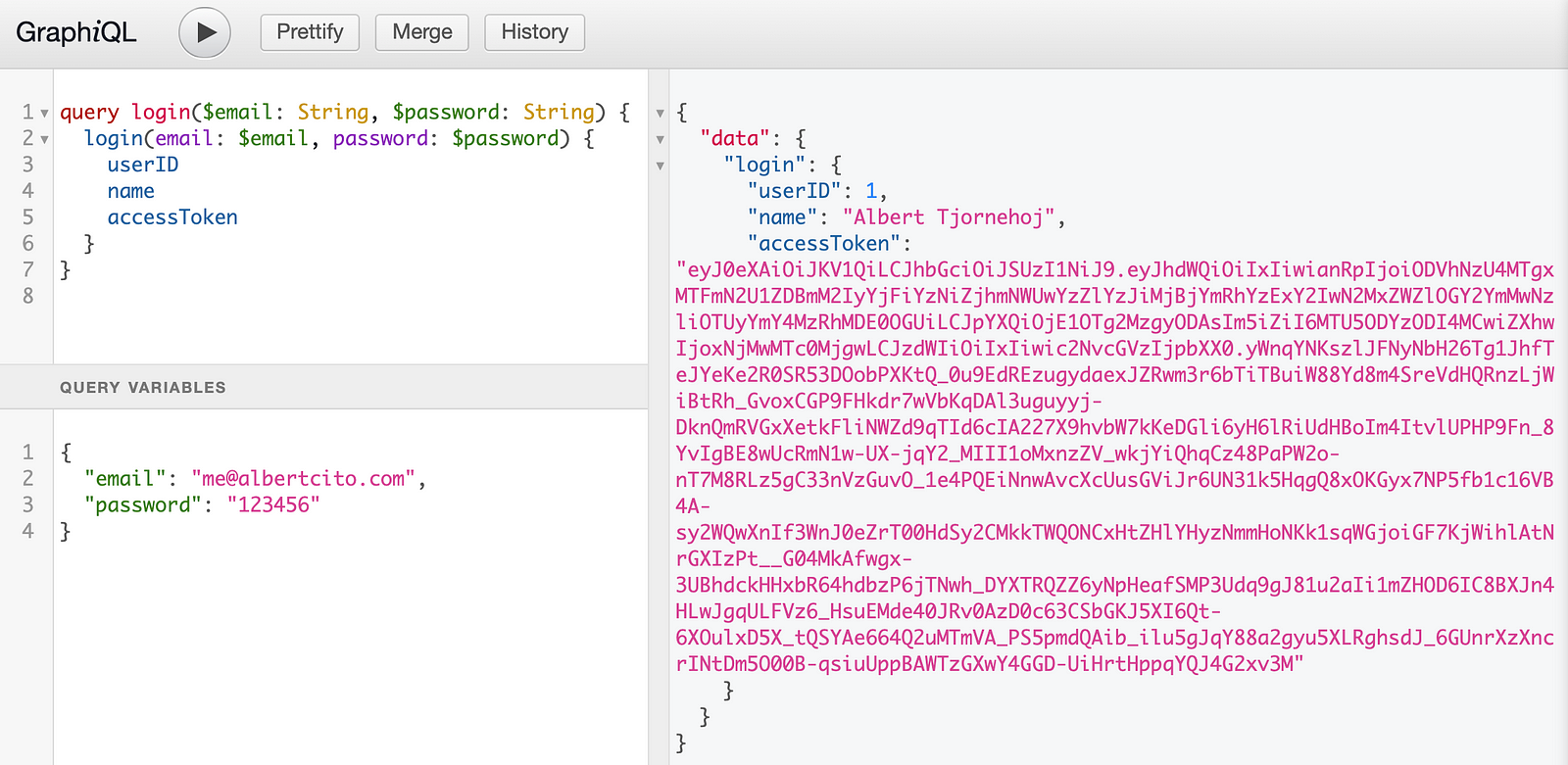
#
1. Install PassportThis example works with Laravel Passport, you can go here to see the instruction to install it.
#
2. Create the UserTypeI'm working with user_id
as primary key for the table user. So, the first step is create the GraphQL UserType.
#
3. Create the login queryThis query receive two params email
and password
. And return the user DB values + accesToken.
#
4. The Login classThis is a basic login class, in the code it was optimized to make it more scalable.
#
5. Add the query and typeIn order to make it available, we need to add the query and the type in the graphql configuration file.
#
6. Run the queryNow you can go to http://127.0.0.1:8000/graphiql/ and run the query and see the DB values in JSON format.
Variables:
This is just a login query. I will post soon how to use it. If you would like to see the code, please go to my github. If you would like to see it live please go here.