Public and private schema with Apollo Server and Express
(you can read a better solution here)
The GraphQL Spec that defines how errors should be handled. That means Apollo Server cannot send 401 server error, each request sent http status 200. Even if you have an authenticate issue, it will return a JSON with http status 200. My plan to handle it is to create two shemas, one private and one public.
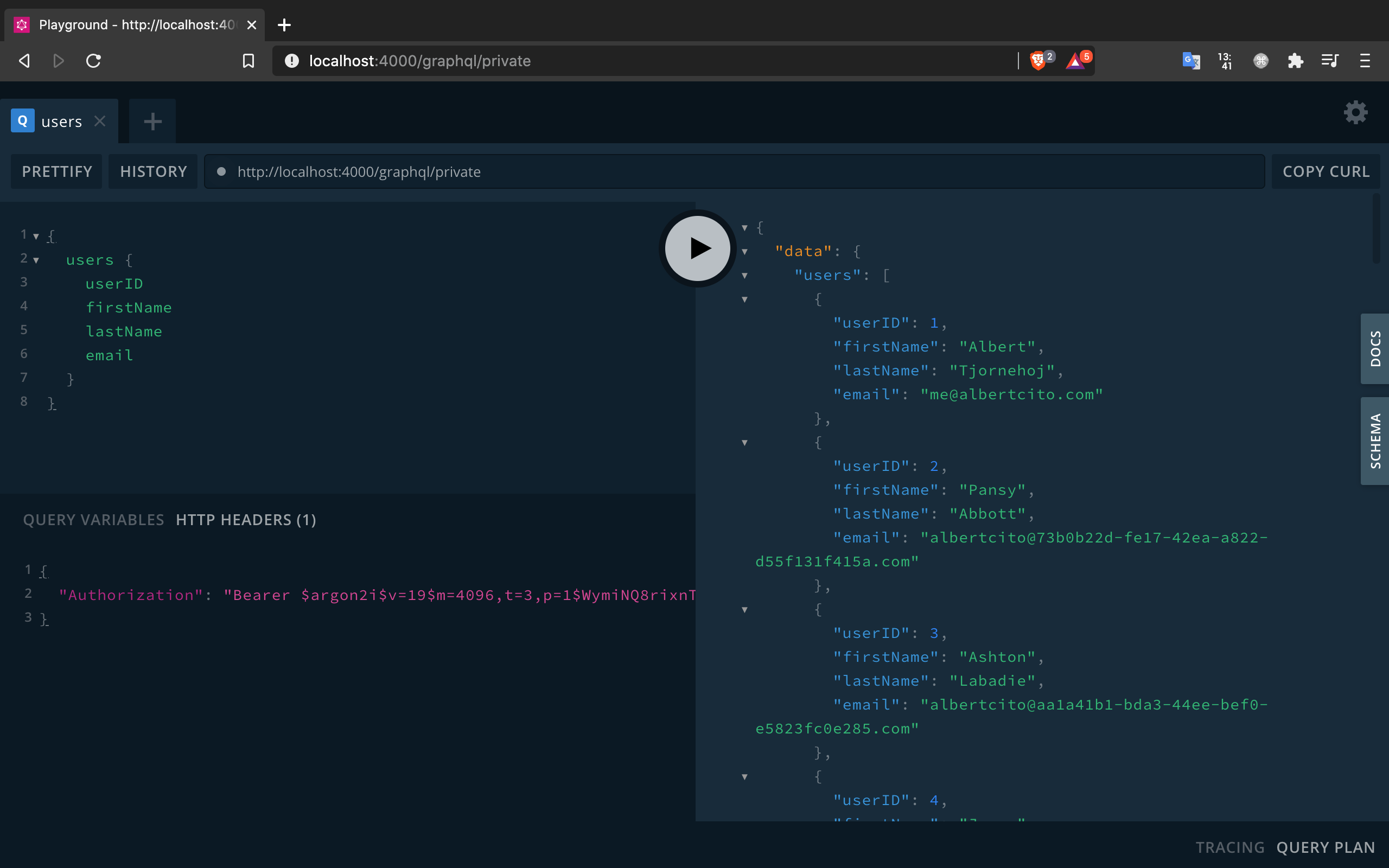
#
Apollo Server middleware problemThe type-graphql middleware will return an http status 200 with a JSON with the UNAUTHENTICATED error code.
#
Create Apollo Server SchemaThis is the private schema. The line 3 has the route for the private queries and mutations. The line 9 has the path name, it means the endpoint will be in locahost:4000/graphql/private
. For the public you have to do the same, change the resolvers path and the path. In my case I have /graphql/private
for the private resolvers and /graphql/public
for the public ones.
#
Create MiddlewareThis is the middleware to block /graphql/private
from unauthenticated users. Unfortunately, the "application-level middleware" in ExpressJS does not show all application information within middleware. But, at least I found a way to see the current request url.
#
Add the GraphQL server and Middleware to ExpressThis is the express app, the private and public Apollo Server was added. And the isAuthMiddleware
to allow authenticated users only for the url graphql/private
.
Finally the solution was to create a private Apollo Server schema, only allow requests for authenticated users. That means that all queries and mutations in that schema will be private. You can see the code in my repo.