Package to use decorators with validatorJS
I just created a package that work with validatorJS and Typescript decorators to validate your classes.
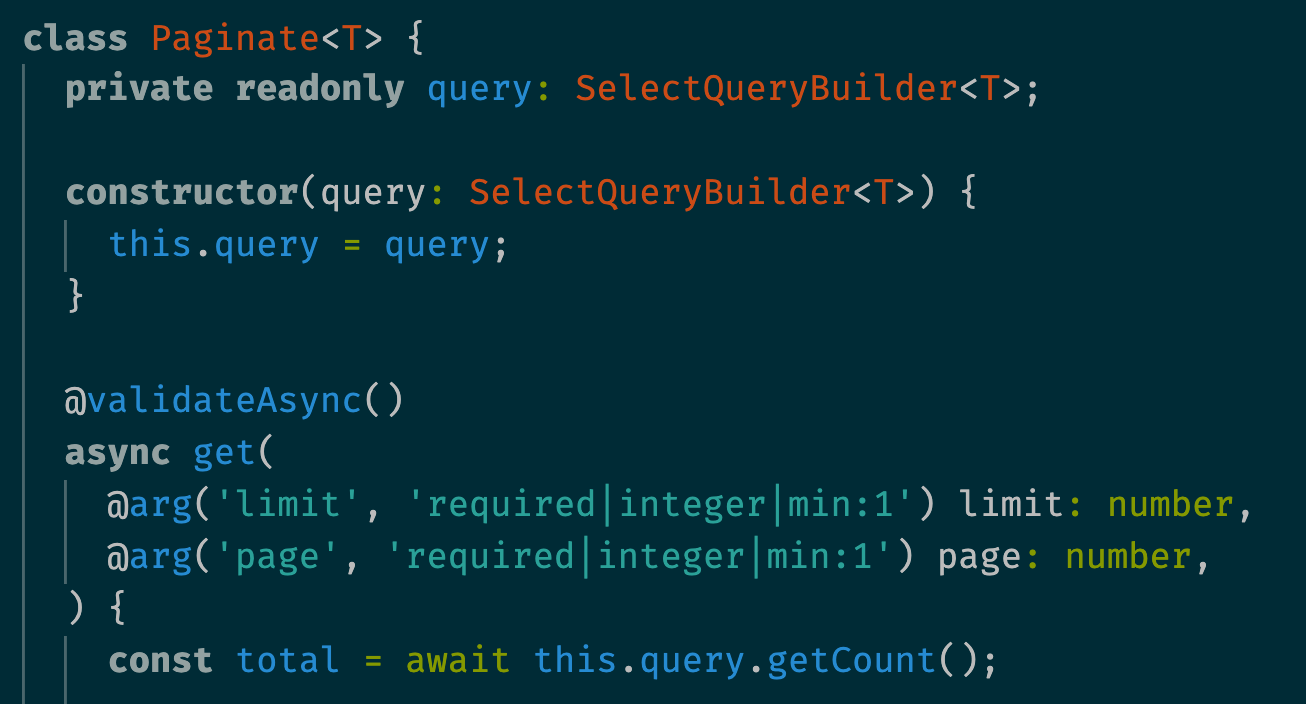
Validation using decorators with validatorjs
#
Validation inside functionI was validating the parameters of the function get in one of this way. It's works but look like the code is unclean.
#
Validation using decorator#
Validate a class functionIt works well, but I decorators make it looks clean and easy to read:
#
Validate a class constructor and propertiesAlso, it works for constructor
or properties:
#
InstallTo install run one of this instructions:
npm install validatorjs-decorator
yarn add validatorjs-decorator
All the rules availables are in the validatorJS repository. The code of validatorjs-decorators package is in my github.