Handle failed server requests with a custom exception in TypeScript
In this post I want to show you how to handle server errors like 404, 500, 403, etc. Also "Network Connection error" and validation errors u others.
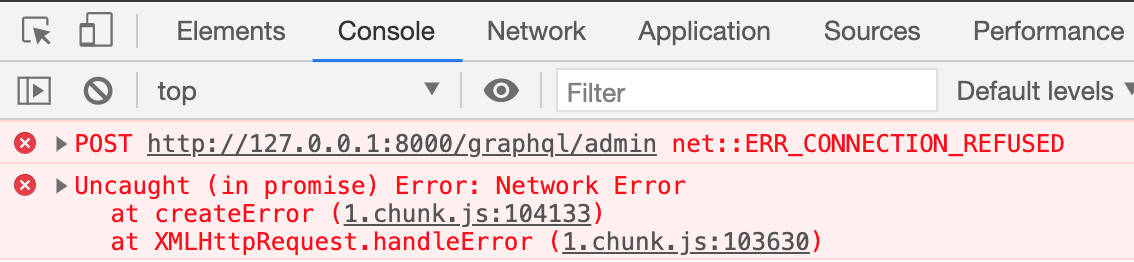
Network Error
If we have a endpoint that send data if the request is right:
If the request fail, it's like that:
#
1. ErrorCodeFormat interfaceThis is a basic interface that cold be used to get the server errors.
So in case of validator error the json format will be:
In case of Network Error or server error, we need to format it:
#
2. ExceptionNow we need to create the Exception that will help us to identify if it's a validation error.
#
3. Api functionThis function get the data from the server and always return a UserFormat or it fail.